Hey! :)
I've a problem I'm thinking about for quite a while with no solution.
This is my project:
The ball is moving left to player (blue). I want that the ball bounces off the player but of course in the right angle (not just turn(180))..
I found no solution because you can turn and move the player so the rotation will change but it should still bounce off the same way althought the rotation changed. How can I manage this?
Thanks :)
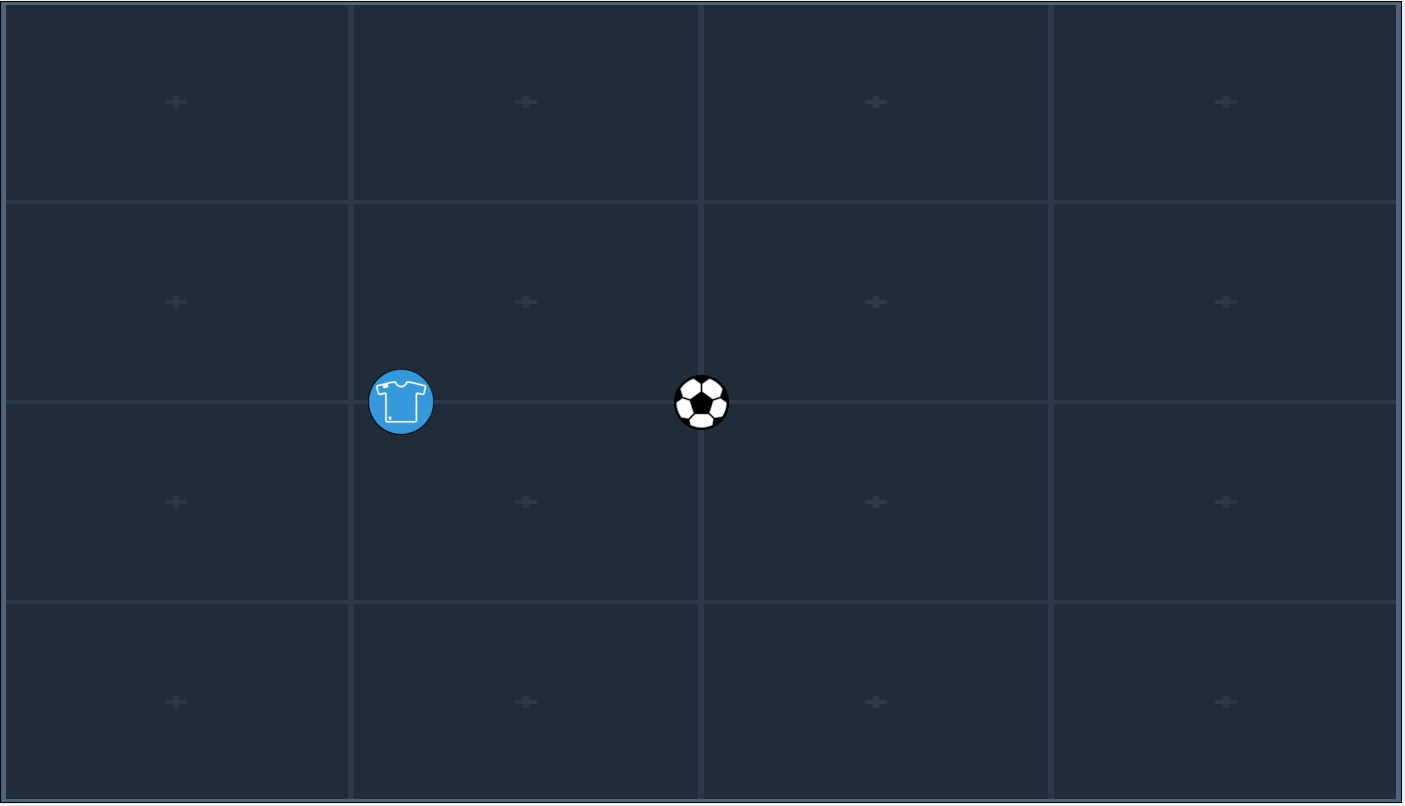